Write a program to print the prime factors of a number. In this C Program example, we are going to create a C program to find the prime factor of the number.
What is a Prime Factor?
Prime factors are factors of a number that is prime.
Example: 3 and 5 are prime factors of 15.
Algorithm of Prime Factor
- Start with including library and declaring variables,
- Performing an operation to get prime factor,
- In the end, print all of them with the appropriate message.
C Program to find Prime Factor of the Number
//factor of a number #include<stdio.h> void main(){ int x,i; printf("enter the number:"); scanf("%d",&x); for(i=2;x>1;i++){ while(x%i==0){ printf("%d ",i); x=x/i; } } }
Explanation of the Source Code
- On-Line no:1 commenting out the topic
- On-Line no:2 including library to take input and to give output
- On-Line no:3 initializing main method with void as its return type
- On-Line no:4 declaring the variables with their datatypes
- On-Line no:5 printing the command to the user for getting the input
- On-Line no:6 using the scanf method to take input
- On-Line no:7 now comes to the logic part to iterate the condition again and again the loop starts with 2 and runs until the condition given became false with increment each time
- On-Line no:8 using the while loop to check the condition again and again until it became false
- On-Line no:9 printing the prime factor we get from the logic
- On-Line no:10 updating the user given value for iteration again
- In the end, we close the scopes of the various method and functions we had opened earlier
Output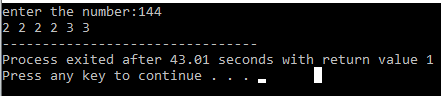
In this way, we learned how to create a program in C to find the prime factor of the number.