In this tutorial, we will learn about the Components of both functional and class-based components, how the rendering of Components takes place. We will learn about the use of props in the component and will learn how they help us to modify components.
What are the components in ReactJS?
Components are building block of React, they help to breakdown the UI into smaller pieces, these pieces have specific and clear responsibilities and well define faces. And we can work on these tiny pieces of the app without affecting the whole app. They encourage us to build applications using composition instead of inheritance. Conceptually they are nothing but the JavaScript functions, they take some input values passed and return REACT elements.
Advantages of Components
- Reusable → Single components can be used in multiple places without rewriting the same piece of code.
- Versatile → We can pass on different properties/data and use same code in many different ways.
- Composition → React uses composition to build user interfaces. Instead of extending base components to add more UI or behaviour, we compose elements in different ways using nesting and props.
How to create Functional Components in ReactJS
Steps:-
- Create an individual file in the src directory to store component
- Create a file Header.js to create a Header component.
- Import React and write a plane JavaScript function returning a JSX term.
- Export the functions and retrieve in App.js file and renders the component in it.
Header.js
import React from 'react'; function Header() { return( <h1>Welcome, This is React Component</h1> ) } export default Header;
App.js
import React from 'react'; import './App.css'; import Header from './Components/Header'; function App() { return ( <div> <Header/> </div> ) }; export default App;
OutPut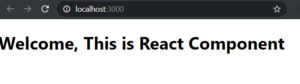
Real-Life Scenario of Components and Props
In real life or production websites, a single component can be used in multiple parts of a web app. This can cause re-writing of the same code, but in React we create the components for the sole purpose of reusability of the same piece of code without re-writing it. For example Navbar in any website, Navbar is common throughout any page just like the home page or Aboutus page, here we can create a component of the navbar and can use to render in multiple pages. And as we have to use component we can make use of props to pass as they can load with the different property set but the same component in overall. This is How components and props are used.
What Are Props in ReactJS?
Props are the property of any component that can be passed to the component as an argument is passed to the function or the attribute is passed to the HTML tag.
We send props to the components using the attribute syntax of the HTML. But while defining the components we must pass the argument (props) in the function so that we can access the property in that component.
Advantages
- Props are the reason the components can be reusable as by passing different argument we can change the output of the component
- Props can pass data to the different components as a parameter.
- We can store the data in the temporary variables and then can pass as props. (Used while fetching the data)
How Props Work in ReactJS
- In the Components, folder create a file Welcome.js
- Import React and create a JavaScript function and pass props as the parameter.
- Return a JSX term and use props.name to display the passing name and export.
- In App.js retrieve the Component and call with passing the name attribute in it.
Welcome.js
import React from 'react'; function Welcome(props) { return( <h1>Welcome to {props.name}</h1> ) } export default Welcome;
App.js
import React from 'react'; import './App.css'; import Header from './Components/Header'; import Welcome from './Components/Welcome'; function App() { return ( <div> <Header /> <Welcome name = 'codebun' /> <Welcome name = 'codedec' /> </div> ) }; export default App;
OutPut:-
Note: – The header component is used from the above example and here we use the Welcome function twice but to tell two different things this makes the components reusable.